PyCharm is an Integrated Development Environment (IDE) specially used for Python programming language. It is cross-platform available for Linux, Mac and Windows, and developed by JetBrains and offers two versions community edition and professional edition. It provides code analysis, a graphical debugger, an integrated unit tester, integration with version control systems (VCSes), and supports web development with Django.
The Community Edition is released under the Apache License, and the Professional Edition released under a proprietary license - this has extra features. Both editions let you write neat and maintainable code while the IDE helps you keep quality under control with PEP8 checks, testing assistance, smart refactorings, and a host of inspections. PyCharm is designed by programmers, for programmers, to provide all the tools you need for productive Python development.
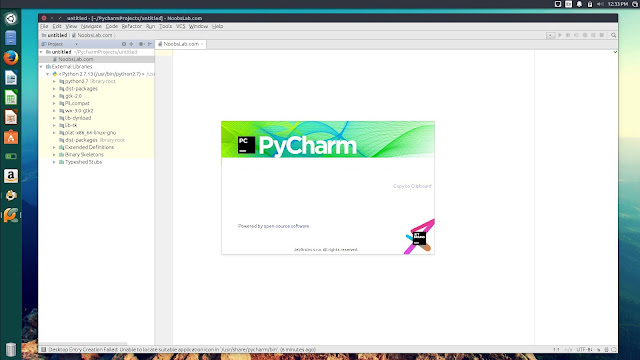
Features:
To install PyCharm Community and Professional edition in Ubuntu/Linux Mint open Terminal (Press Ctrl+Alt+T) and copy the following commands in the terminal:
For Pycharm Professional or Educational use these command:
To install PyCharm Community and Professional edition in Ubuntu/Linux Mint open Terminal (Press Ctrl+Alt+T) and copy the following commands in the terminal:
For Pycharm Professional run this command:
That's it
The Community Edition is released under the Apache License, and the Professional Edition released under a proprietary license - this has extra features. Both editions let you write neat and maintainable code while the IDE helps you keep quality under control with PEP8 checks, testing assistance, smart refactorings, and a host of inspections. PyCharm is designed by programmers, for programmers, to provide all the tools you need for productive Python development.
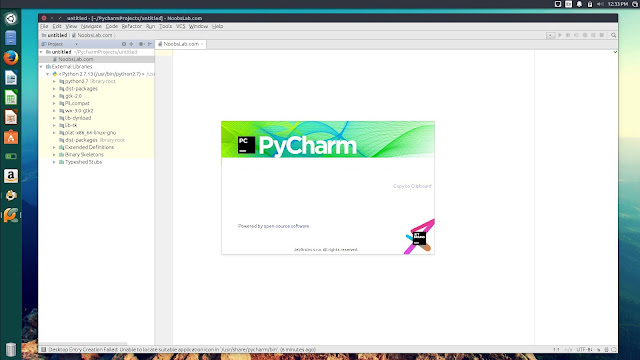
Features:
- Coding Assistance and Analysis, with code completion, syntax and error highlighting, linter integration, and quick fixes
- Project and Code Navigation: specialized project views, file structure views and quick jumping between files, classes, methods and usages
- Python Refactoring: including rename, extract method, introduce variable, introduce constant, pull up, push down and others
- Support for web frameworks: Django, web2py and Flask
- Integrated Python Debugger
- Integrated Unit Testing, with line-by-line code coverage
- Google App Engine Python Development
- Version Control Integration: unified user interface for Mercurial, Git, Subversion, Perforce and CVS with changelists and merge
- PyCharm 50+ IDE plugins of different nature, including support for additional VCS
Install via Snap package
Available for Ubuntu 18.10 Cosmic/18.04 Bionic/16.04 Xenial/Linux Mint 19.x/18/and other related Ubuntu derivativesTo install PyCharm Community and Professional edition in Ubuntu/Linux Mint open Terminal (Press Ctrl+Alt+T) and copy the following commands in the terminal:
For Pycharm Professional or Educational use these command:
Install via PPA
Available for Ubuntu 18.10 Cosmic/18.04 Bionic/16.04 Xenial/14.04 Trusty/Linux Mint 19.x/18/17/and other related Ubuntu derivativesTo install PyCharm Community and Professional edition in Ubuntu/Linux Mint open Terminal (Press Ctrl+Alt+T) and copy the following commands in the terminal:
For Pycharm Professional run this command: